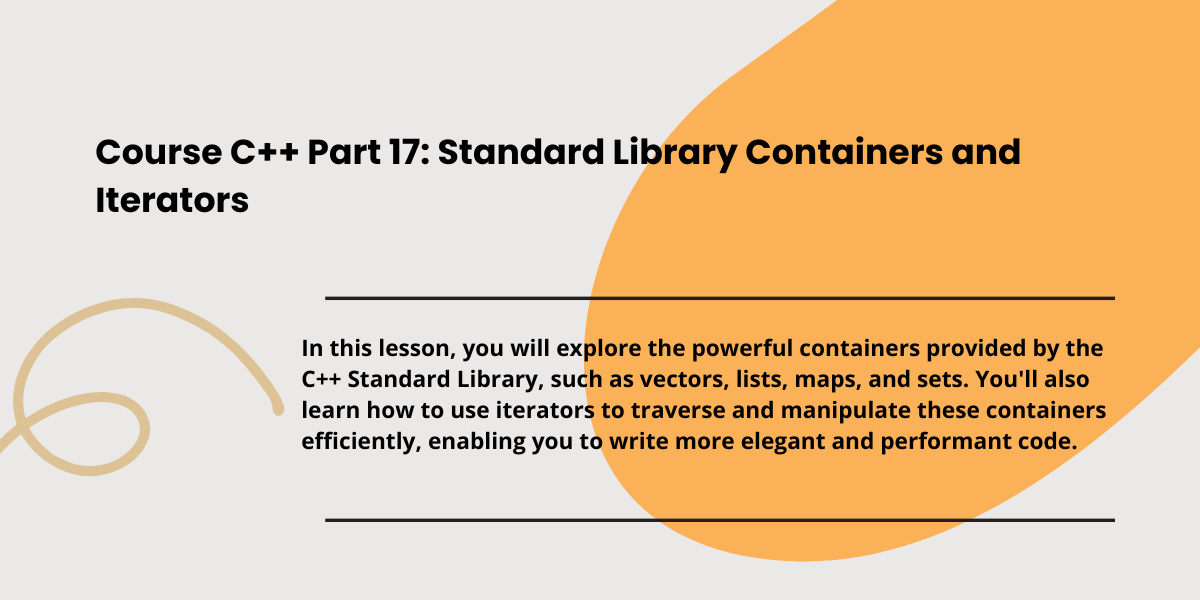
Course C++ Part 17: Exploring Standard Library Containers and Iterators
Course Description
In this comprehensive lesson, you’ll gain an in-depth understanding of the Standard Library Containers and Iterators in C++. These essential tools simplify data management and enhance your ability to write more efficient, readable, and maintainable code. You’ll start by exploring a variety of container types—such as vectors, lists, sets, and maps—learning their unique characteristics, advantages, and best-use scenarios.
We’ll dive into the concept of iterators, which are key to traversing container elements in a consistent and flexible manner, regardless of the underlying container type. You’ll learn about the different iterator types (input, output, forward, bidirectional, and random access) and how to use them effectively in your code.
The lesson also covers important operations like inserting, deleting, and modifying elements within containers using iterators, as well as the practical aspects of combining iterators with algorithms from the Standard Library. By mastering containers and iterators, you’ll be able to optimize your C++ programs for performance and scalability, improving your ability to handle large datasets and complex operations with ease.
By the end of this lesson, you’ll be well-equipped to leverage the full power of C++ containers and iterators, making your code more efficient, modular, and easier to maintain in real-world applications.
Course Curriculum
- 001. Lesson 17 Overview—Exploring Parallel Algorithms and Concurrency: An Overview
- 002. Introduction to Parallel Algorithms and Concurrency in C++
- 003. Exploring Standard Library Parallel Algorithms in C++
- 004. Benchmarking Sequential vs. Parallel Sorting Algorithms in C++
- 006. Determining the Right Time to Use Parallel Algorithms in C++
- 007. Understanding Execution Policies in C++ Parallel Algorithms
- 008. Profiling Parallel and Vectorized Operations in C++
- 009. Further Insights into Parallel Algorithms in C++
- 010. Understanding Thread States and Life Cycle in C++
- 011. Using std::jthread to Launch Tasks in C++
- 012. Creating and Defining Tasks for Threads in C++
- 013. Running Tasks with std::jthread in C++
- 014. How std::jthread Resolves Common Threading Issues in C++
- 015. Implementing a Producer-Consumer Model: A First Attempt