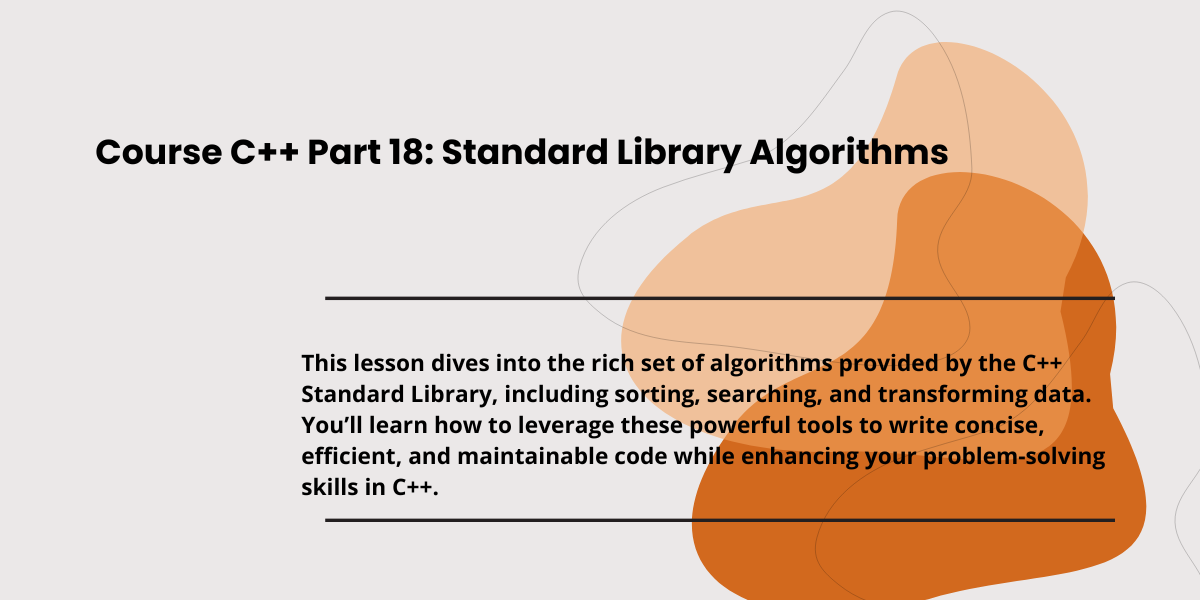
Course C++ Part 18: Mastering Standard Library Algorithms
Course Description
In this lesson, you'll gain an in-depth understanding of C++'s Standard Library algorithms, which are essential tools for solving a wide variety of programming challenges efficiently. You’ll learn how to leverage these algorithms to perform common tasks such as searching, sorting, transforming, and manipulating data with minimal code. The lesson covers key concepts like iterators, predicates, and how to apply these algorithms to different container types, from vectors and lists to maps and sets. You’ll also explore how algorithms can be combined for more complex operations, optimizing both performance and readability of your code. Through practical examples and real-world use cases, you’ll understand how to apply algorithms effectively in different contexts, ensuring your code runs faster and with fewer bugs. By the end of this lesson, you’ll be well-equipped to use the Standard Library algorithms in your projects, allowing you to write more efficient, maintainable, and error-free C++ code. Whether you're working on data processing, game development, or systems programming, these powerful tools will save you time and improve your programming expertise.
Course Curriculum
- 001. C++20 Coroutines Overview: Unlocking the Power of Asynchronous Programming
- 002. Understanding Coroutines in C++20: A Beginner's Guide
- 003. Exploring Coroutine Support Libraries in C++20
- 004. Setting Up the Concurrencpp and Generator Libraries: A Guide to Installing Concurrencpp
- 005. Installing the Concurrencpp Library in Visual Studio with vcpkg
- 006. Installing the Concurrencpp Library with Docker/Linux and vcpkg
- 007. Installing the Concurrencpp and Generator Libraries—Generator Library Setup
- 009. Building a Generator Coroutine with co_yield and the Generator Library—Control Flow Diagram for a Generator Coroutine
- 011. Task Launching with concurrencpp – Using concurrencppruntime for Asynchronous Execution