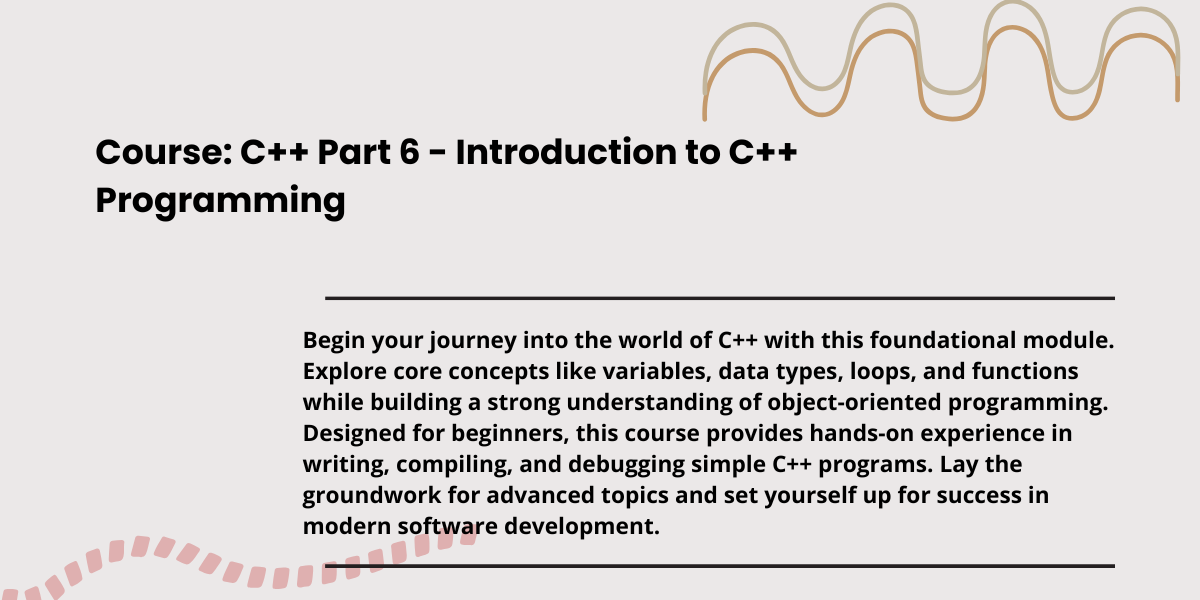
Course C++ Part 6: Introduction to C++ Programming Essentials
Course Description
This course serves as the ideal starting point for anyone looking to learn C++ programming or refresh their existing skills. In this module, you'll explore the foundational concepts that are essential for becoming proficient in C++. The course covers a range of topics, starting with basic syntax, data types, and variables. You'll also dive into control structures like if-else statements and loops, as well as functions, which are crucial building blocks in programming.
Through practical examples and interactive exercises, you'll get hands-on experience with writing and running C++ code. This lesson emphasizes problem-solving techniques and helps you develop a logical approach to writing efficient code. Whether you're new to programming or transitioning from another language, this course will provide you with the knowledge and skills you need to progress further in C++ development.
By the end of this part, you’ll be comfortable with basic programming principles and ready to tackle more advanced topics in C++ programming, making it an essential step in your coding journey.
Course Curriculum
- 001. Exploring Arrays, Vectors, Ranges, and Functional Programming Concepts
- 002. Populating Array Elements Using Loops
- 003. Setting Up Arrays Using Initializer Lists
- 004. Exploring Range-Based for Loops and C++20 Initializer Enhancements
- 005. Using Calculations to Set Array Elements and Exploring constexpr in C++
- 006. Summing Array Elements Using External Iteration in C++
- 007. Visualizing Array Data with a Simple Bar Chart in C++
- 008. Revisiting Die Rolling: Using Array Elements as Counters in C++
- 009. Summarizing Survey Results with Arrays in C++
- 010. Organizing and Finding Data: Sorting and Searching Arrays in C++
- 011. Working with Multidimensional Arrays in C++
- 012. Introduction to Functional Programming Concepts in C++
- 013. Introduction to Functional-Style Programming: Functional Reduction with accumulate