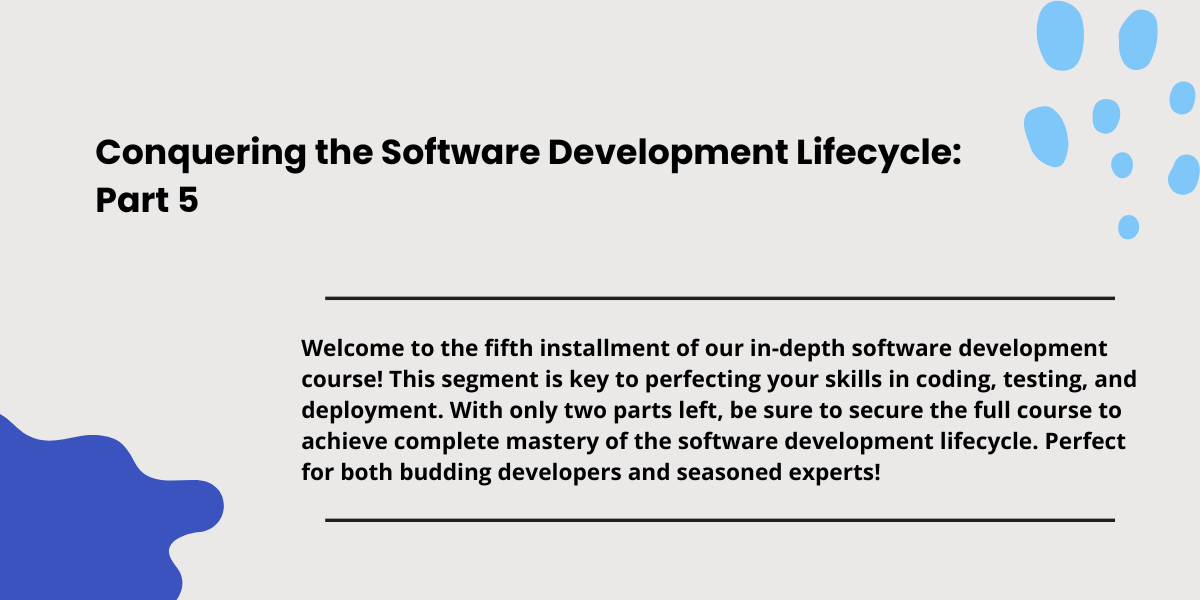
Course C++ Part 13: Crafting Custom Classes for Efficient C++ Programming
Course Description
In this in-depth lesson, you'll master the essential techniques for creating custom classes in C++ to solve real-world programming challenges. You'll start by learning how to define and implement classes, understanding how to organize related data and behavior into cohesive structures. We’ll cover key components such as constructors, destructors, member functions, and operator overloading, allowing you to build versatile and maintainable classes. Additionally, we’ll discuss important topics like encapsulation, data hiding, and the significance of access modifiers (private, public, protected) in controlling access to class members. You’ll also learn how to implement dynamic memory management within classes and the proper use of pointers and references. By the end of the course, you’ll have the ability to design flexible, efficient, and reusable classes tailored to your project’s needs, laying the groundwork for advanced object-oriented programming in C++. Whether you're developing complex data structures, working with real-world systems, or simply improving your coding practices, this lesson will equip you with the knowledge and skills to write cleaner, more efficient C++ code.
Course Curriculum
- 001. Lesson 13 Overview: Mastering Standard Library Containers and Iterators in C++
- 002. Getting Started with Standard Library Containers and Iterators in C++
- 004. Exploring Common Nested Types in Sequence and Associative Containers in C++
- 005. Key Member and Non-Member Functions in C++ Containers
- 006. Understanding the Requirements for Container Elements in C++
- 007. Mastering Iterators in C++ for Efficient Container Navigation
- 008. Leveraging istream and ostream Iterators for Efficient Input and Output in C++
- 009. Understanding Iterator Categories in C++ for Optimized Container Navigation
- 010. Iterator Support Across C++ Containers: Enhancing Flexibility and Efficiency
- 011. Exploring Predefined Iterator Type Names in C++ for Simplified Code
- 012. Mastering Iterator Operators in C++ for Efficient Data Traversal
- 013. Exploring Sequence Containers in C++: A Deep Dive
- 014. Mastering Vectors and Iterators in C++: Efficient Data Handling
- 015. Exploring Vector Element Manipulation Functions in C++