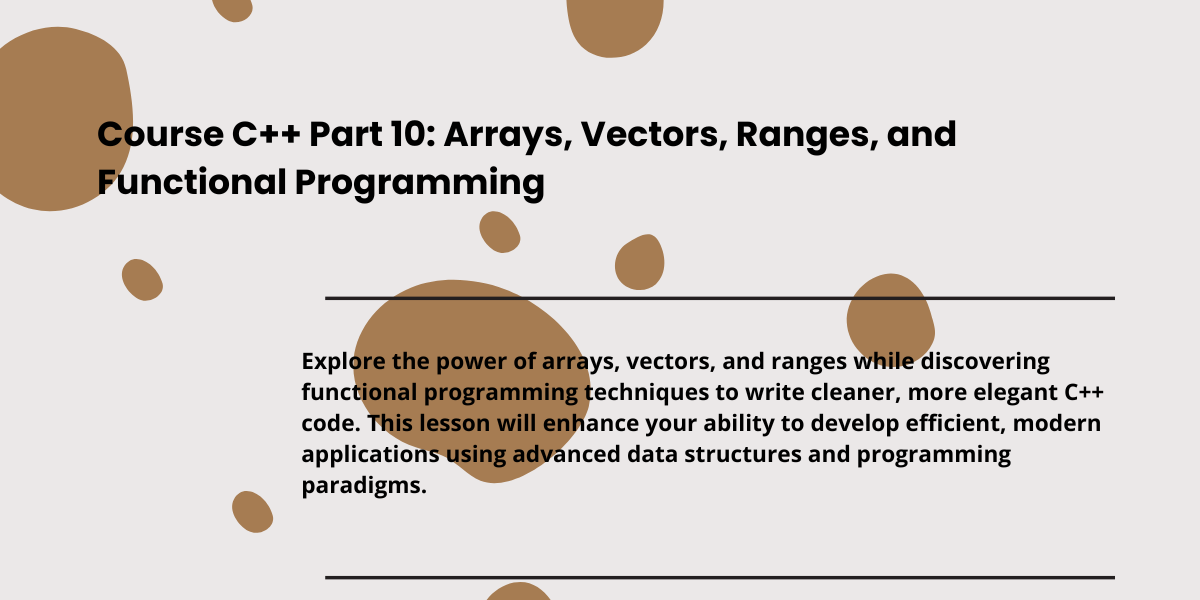
C++ Course Part 10: Working with Arrays, Vectors, Ranges, and Functional Programming
Course Description
In this section of the C++ course, you'll delve into some of the most fundamental data structures in C++: arrays and vectors. You'll learn how to store, access, and manipulate data using these structures effectively. In addition, you'll explore C++'s powerful range-based features, which simplify working with sequences of data, enabling you to write more concise and expressive code.
You'll also be introduced to functional-style programming, a paradigm that emphasizes the use of functions as first-class citizens. This approach encourages immutability, higher-order functions, and declarative programming techniques. By mastering these concepts, you'll learn how to write cleaner, more maintainable, and efficient code.
Whether you're processing large datasets, performing complex transformations, or simply looking to improve your coding style, this section will equip you with the tools to handle data more effectively. You'll be able to apply advanced programming paradigms, improving the readability and performance of your C++ projects.
Course Curriculum
- 001. Lesson 10 Overview: Object-Oriented Programming - Inheritance and Polymorphism
- 002. Parent and Child Classes in OOP
- 003. Understanding the Connection Between Parent and Child Classesс
- 004. Designing and Implementing a SalariedEmployee Class
- 005. Building an Inheritance Hierarchy: SalariedEmployee and SalariedCommissionEmployee
- 006. Working with Constructors and Destructors in Inherited Classes
- 007. Understanding Object Relationships in an Inheritance Hierarchy
- 008. Calling Base-Class Methods from Derived-Class Objects
- 009. Pointing Derived-Class Pointers to Base-Class Objects
- 010. Invoking Derived-Class Functions through Base-Class Pointers
- 011. Understanding Virtual Functions in C++
- 012. Avoid Calling Virtual Functions in Constructors and Destructors
- 013. Working with Virtual Destructors in C++
- 014. Using Final for Member Functions and Classes in C++