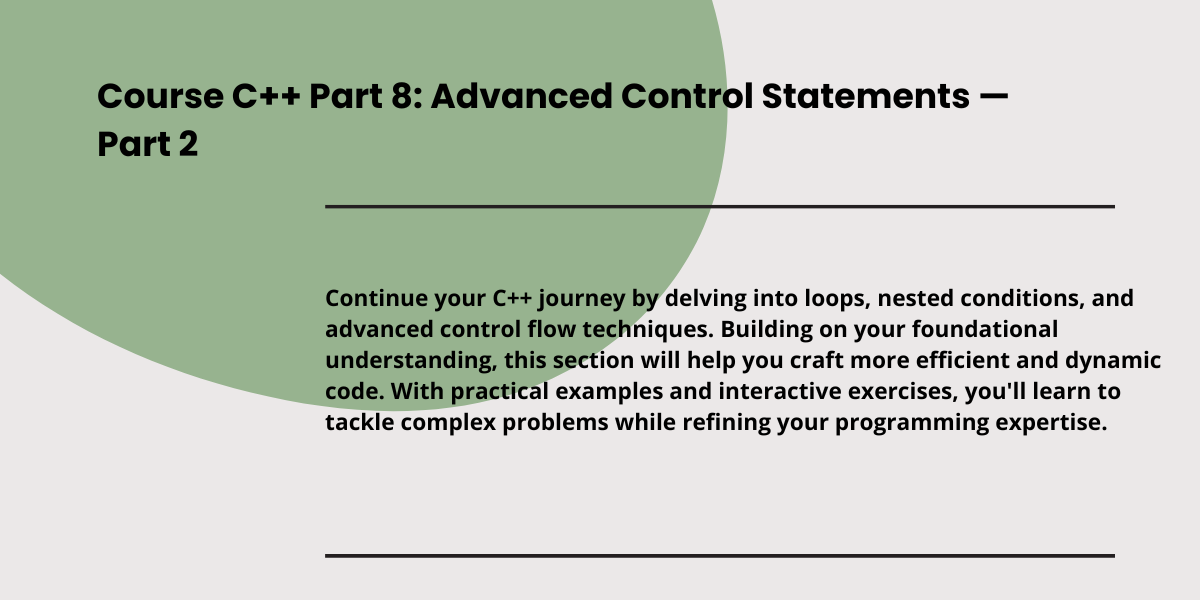
C++ Course Part 8: Introduction to Control Flow Statements - Part 1
Course Description
In this lesson, we thoroughly explore the essential control flow statements in C++, which are critical for managing the execution flow of your programs. We begin by covering conditional statements like if, else, and switch, which allow you to make decisions based on specific conditions. You'll also learn how to implement various loop constructs such as for, while, and do-while, enabling you to repeat actions and iterate over data effectively. The lesson includes detailed examples that demonstrate how these statements are applied in different scenarios, from basic conditional checks to more complex decision-making processes. Additionally, we highlight the practical use cases for each statement, helping you understand when and why to use them in real-world applications. By the end of this part, you'll not only grasp the foundational control flow concepts but also be well-equipped to build more sophisticated algorithms and logic in C++, setting the stage for more advanced programming techniques.
Course Curriculum
- 001. Lesson 7 Overview: Minimizing the Use of Pointers in Modern C++
- 002. Introduction to Reducing Pointer Usage: When Pointers Are Necessary and C++20 Alternatives
- 003. Declaring and Initializing Pointer Variables in C++
- 004. Understanding Pointer Operators in C++
- 005. Passing by Reference Using Pointers in C++
- 007. Converting Built-in Arrays to std::array in C++20 Using to_array
- 008. Using const with Pointers and Their Target Data in C++
- 009. Understanding the sizeof Operator in C++
- 010. Mastering Pointer Expressions and Arithmetic in C++
- 011. C++20 Spans: A Case Study on Views of Contiguous Container Elements
- 012. An Introduction to Pointer-Based Strings in C++
- 013. Working with Command-Line Arguments in C++
- 014. Exploring C++20’s to_array Function in Depth
- 015. Exploring Advanced Pointer Concepts in C++