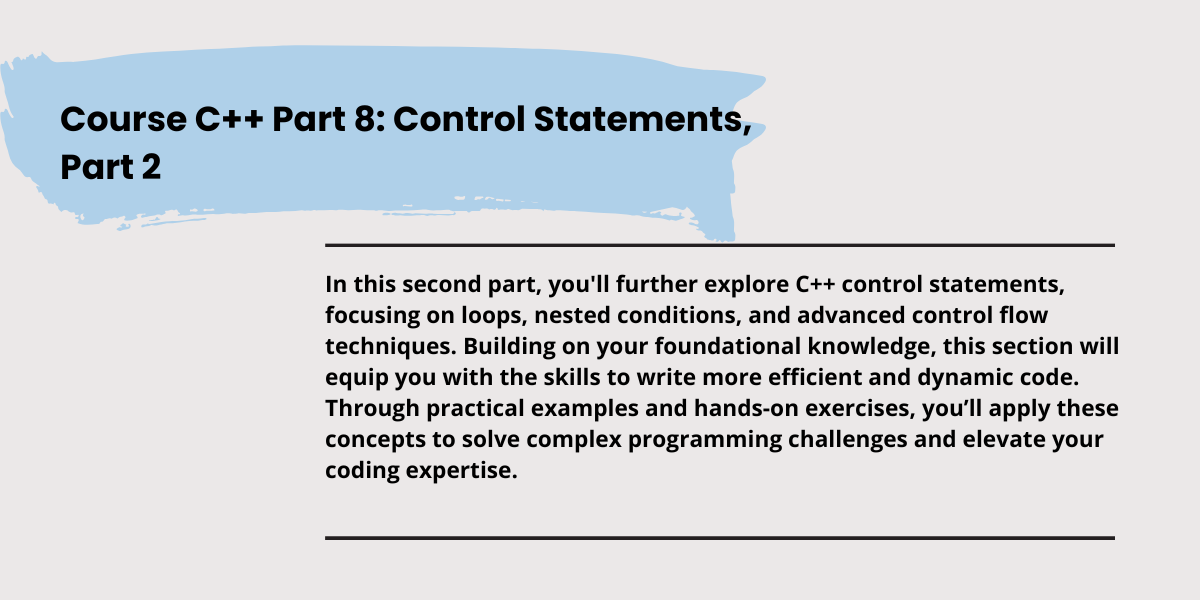
C++ Course Chapter 8: Advanced Control Flow
Course Description
In this chapter, we take a comprehensive look at control flow mechanisms in C++. These tools are critical for creating programs that can make decisions and repeat operations based on specific conditions. We’ll focus on advanced structures such as selection statements (if, else, switch) and loops (for, while, do-while), examining their syntax, functionality, and practical applications.
You’ll also explore how to utilize special keywords like break and continue to fine-tune the behavior of your loops and conditional statements. Through hands-on examples and exercises, you’ll gain a deeper understanding of how to implement branching logic to solve complex programming challenges.
By the end of this chapter, you’ll be equipped with the skills to write efficient, flexible, and maintainable code. Whether you’re managing simple conditions or designing intricate control structures, this knowledge will be an essential foundation for tackling real-world programming problems with confidence.
Course Curriculum
- 001. Lesson 8: Exploring Strings, File Handling, and Regular Expressions
- 002. String Manipulation: Assignment and Combining
- 003. String Comparison Techniques
- 004. Working with Substrings
- 005. Exchanging String Values
- 006. Properties of Strings
- 007. Locating Substrings and Characters within a String
- 008. Locating Substrings and Characters within a String
- 009. Adding Characters to a String
- 010. Converting Between Strings and Numeric Types in C++11
- 011. C++17 String View: An Introduction
- 012. Building a Sequential Data File
- 013. Extracting Data from a Sequential File
- 014. C++14 Handling Quoted Text: Reading and Writing
- 015. String Stream Handling: Using ostringstream
- 017. Working with Raw String Literals
- 018. Reading CSV File Data with RapidCSV
- 019. Exploring and Analyzing the Titanic Dataset, Part 1
- 023. Objects and Case Study: Introduction to Regular Expressions
- 024. Matching Entire Strings to Specific Patterns