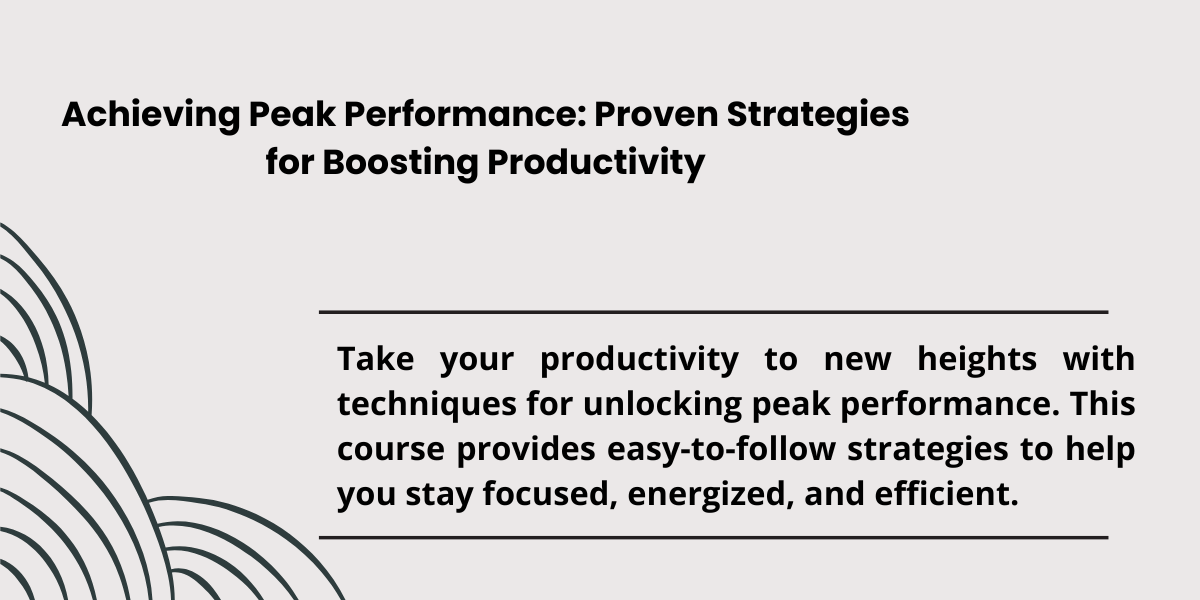
C++ Part 14: Mastering Object-Oriented Programming
Course Description
In this section of the course, you'll dive deep into the foundational concepts of Object-Oriented Programming (OOP) in C++. Object-Oriented Programming is a paradigm that allows you to structure and organize your code in a way that mirrors real-world systems, making it easier to design, maintain, and scale complex software.
You will begin by learning about classes and objects, the building blocks of OOP. Classes serve as blueprints for creating objects, while objects are instances of these classes. You’ll gain hands-on experience creating your own classes, setting properties, and defining behaviors through methods, allowing you to model real-world entities in code.
Next, you will study the principle of inheritance, which enables one class to inherit properties and methods from another, promoting code reuse and establishing relationships between different classes. This will lead to exploring polymorphism, a powerful concept that allows objects to take many forms, enabling you to write more flexible and dynamic code. You'll learn how to override methods in derived classes and how to use virtual functions to implement dynamic method dispatch, making your code more extensible.
Additionally, the concept of encapsulation will be covered, which focuses on restricting access to certain details of an object's internal state, exposing only what is necessary. This is crucial for data protection and ensuring that objects interact in a controlled way, maintaining the integrity of the system.
Throughout this lesson, you will gain practical skills for applying these concepts in real-world applications, allowing you to build sophisticated and modular C++ programs. By mastering OOP principles, you will be able to design flexible, scalable software solutions that are easier to maintain, debug, and extend as your projects grow in complexity. This will enhance your ability to write clean, efficient, and maintainable code, setting the foundation for more advanced programming techniques in C++.
Course Curriculum
- 001. Lesson 14 Overview – Exploring Standard Library Algorithms in C++
- 002. C++20 Concepts: Understanding Algorithm Requirements
- 003. Using Lambdas with Algorithms in C++
- 004. Advanced Techniques with Lambdas and Algorithms in C++
- 005. Using fill, fill_n, generate, and generate_n in C++
- 006. Equality Assessment, Mismatch Identification, and Lexical Sequence Comparison
- 007. Element Removal and Conditional Filtering Techniques
- 008. Element Replacement and Conditional Copying Strategies
- 009. Randomization, Element Counting, and Extremum Detection Algorithms
- 010. Algorithms for Data Searching and Order Sorting
- 011. Element Swapping and Range Exchange Techniques
- 012. Advanced Copying, Merging, Filtering, and Reversal Techniques
- 013. In-Place Merging, Unique Copying, and Reversal Copying Operations
- 014. Set Manipulation Techniques
- 015. Boundary Search and Range Identification Methods